Retrieving Smart Discovery data
Smart Discovery data represents the raw data content of a Smart Discovery. The data contains the full details of the Smart Discovery collections that together make up the Smart Discovery. The settings for generating the data are defined in a Smart Discovery strategy.
To build the Smart Discovery for display on a web page, you must first retrieve the corresponding Smart Discovery data and then populate the Smart Discovery web page element with that data. You retrieve the data based on the Smart Discovery strategy responsible for generating the data.
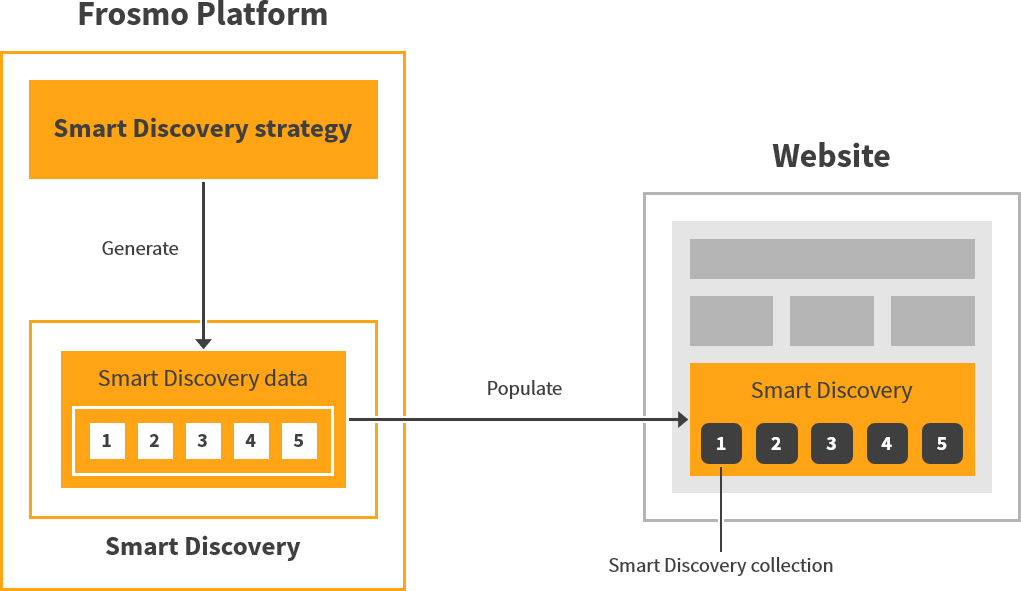
You have two API options for retrieving Smart Discovery data:
-
Frosmo Core fetch function for Smart Discoveries. Use this option if you're retrieving the data on a website that has a basic feature setup. For more information, see Page context and Using the fetch function.
-
Smart Discovery API. Use this option if you're retrieving the data in a native mobile application or a server-side application, or on a website that does not have a basic feature setup. For more information, see Page context and the Smart Discovery API guide.
If you use a modification to display the Smart Discovery on a website:
-
Retrieve the Smart Discovery data and build the Smart Discovery web page element in a template.
-
Retrieve and process the data in the template's content prerenderer.
Page context
To return the correct Smart Discovery data for a web page, the Frosmo Platform requires contextual information about the page. This information is the page context.
The page context describes the relevant aspects of the page based on page type. For example, a brand page requires a brand name as context, while a category page requires one or more category names as context. The API must provide the page context dynamically at runtime based on the specifics of the current page.
The Frosmo Core fetch function can automatically handle the page context on any website that has a basic feature setup. The function requires the necessary page context to be always available on a page. If your site has a basic feature setup, the Frosmo Platform programmatically sets the correct page context on page load, allowing the fetch function to work. If your site does not have a basic feature setup, or if you're retrieving Smart Discovery data from an external application, where Frosmo Core is not available, you cannot use the fetch function.
You need to worry about the page context only if you're using the Smart Discovery API. For more information, see the context
query parameter in the Smart Discovery API reference.
Using the fetch function
To retrieve the data for a Smart Discovery strategy using the Frosmo Core fetch function:
-
Call the
frosmo.easy.smartDiscovery.fetch()
function with the Smart Discovery strategy ID as a string parameter. Make sure that the page where you call the function matches the page type defined for the strategy. -
Extract the data from the response.
You can now use the data in a Smart Discovery web page element.
For a practical example of retrieving and using Smart Discovery data in a template, see Example: Creating a Smart Discovery template.
frosmo.easy.smartDiscovery.fetch()
The frosmo.easy.smartDiscovery.fetch()
function returns a Frosmo Core promise object (compatible with a Promise
) that is either resolved with a success object containing the requested Smart Discovery data or rejected with an error object.
The function requires that the necessary page context is available and that the context matches the page type defined for the Smart Discovery strategy. If the correct page context is not available, the promise fails to resolve. For example, if the page type of the strategy is category
, the function expects to be called on a category page that has a category page context available.
The function uses the Smart Discovery API to retrieve Smart Discovery data.
Request
frosmo.easy.smartDiscovery.fetch('strategy-id')
.then(function (response) {
console.log(response.data);
})
.catch(function (error) {
console.error(error);
});
frosmo.easy.smartDiscovery.fetch('strategy-id', { debug: true, limit: 10, timeout: 10000 })
.then(function (response) {
console.log(response.data);
console.log(response.debug);
})
.catch(function (error) {
console.error(error);
});
Parameters
The following table describes the parameters you can define for the frosmo.easy.smartDiscovery.fetch()
function.
Parameter | Description | Type | Role | Example |
---|---|---|---|---|
| ID of the Smart Discovery strategy whose data to retrieve. | String | Required |
|
| Options for configuring the fetch operation and response. For more information about the options, see the following table. | Object | Optional |
|
Property | Description | Type | Role | Example |
---|---|---|---|---|
| Define whether to return debugging information about the request. warning Use the debugging information only for development and testing purposes. Do not use the information in production, since Frosmo does not guarantee that the information structure and content will remain the same. The possible values are:
The default value is For more information about what the debugging information contains, see the Smart Discovery API reference. | Boolean | Optional |
|
| Maximum number of collections to return in the Smart Discovery data. The function returns the top collections by relevance (as determined by item popularity and the visitor's affinities). The minimum value is The default value is | Number | Optional |
|
| Time in milliseconds that the function waits for the Smart Discovery API to return the requested data. If the API does not return data in the specified time, the function rejects the promise. The default value is undefined, meaning the function waits forever. | Number | Optional |
|
Response
Success
On a success, the frosmo.easy.smartDiscovery.fetch()
function returns a JSON object containing the requested Smart Discovery data.
The success object contains the following root properties:
-
data
: Smart Discovery data as an array of objects, where each object contains the details of a single Smart Discovery. -
debug
: Object containing the debugging information. This property is included only when thedebug
option is set totrue
in the request.
For more information about the contents of the success object, see the Smart Discovery API reference.
Error
On an error, the frosmo.easy.smartDiscovery.fetch()
function returns an object containing information about the error.
The error object contains the following properties:
-
errorThrown
: Object containing information about the error. -
textStatus
: Value of thexhr.statusText
property. -
xhr
:XMLHttpRequest
object containing information about the HTTP request triggered by the function.