Setting the Frosmo ID cookie from a web server
If your website visitor is on a Safari browser, and if your site uses shared context, the Frosmo Platform stores the visitor's Frosmo ID in a first-party cookie called frosmo_uid
with a 365-day expiration. However, since the cookie is set by a script, Safari deletes the cookie if the visitor does not directly interact with the site in 7 days of browser use, regardless of the cookie's expiration date. If the cookie was instead set by your web server, Safari would allow for a longer grace period.
Example: A new visitor using Safari arrives on your site on 2023-12-12, and the Frosmo Platform stores their visitor ID in a frosmo_uid
cookie set to expire on 2024-12-12. Over the next 10 days, the visitor uses the browser on 6 separate days, but they do not interact with your site once. On 2023-12-23, the visitor uses the browser again. If they do not interact with your site on this seventh day of use, Safari deletes the cookie. If they do interact with the site on 2023-12-23, the deletion counter resets.
On Apple mobile devices, all browsers currently use the same WebKit engine as Safari and therefore apply the same 7-day cookie deletion limit. This means that even if a visitor uses a browser other than Safari on their Apple mobile device, they are effectively using Safari.
If you have a lot of visitors who use Safari, and if your site uses shared context, the 7-day cookie deletion limit results in an inflated number of new visitors, skewed site statistics, and shallow personalization experiences.
To sidestep this problem, configure your web server to set its own Frosmo ID cookie.
Setting the Frosmo ID cookie from your server
To configure your web server to set its own Frosmo ID cookie:
-
Contact Frosmo support, and request that visitor ID cookie support is enabled for your site. When this support is enabled, the Frosmo Platform always:
-
Stores a visitor's Frosmo ID in the
frosmo_uid
cookie. -
Synchronizes the
frosmo_uid
cookie to match the value of the Frosmo ID cookie set by your server. This makes your server's cookie the source of truth for each visitor's Frosmo ID.
-
-
Update your web server configuration:
-
Check whether a request from a browser contains the
frosmo_uid
cookie. -
If a request contains the
frosmo_uid
cookie, set the following new cookie in the response to the browser:-
Name:
frosmo_uid_server
-
Value: Same as for the
frosmo_uid
cookie -
Expiry: In 1 year
noteAlways set the
frosmo_uid_server
cookie, even if it's already included in the request, as this will incrementally extended the cookie's expiration date.noteDo not set the
HttpOnly
attribute for the cookie as that will prevent the Frosmo Platform from reading the cookie. -
-
Your site now uses a persistent cookie for the Frosmo ID that also visible to your server-side applications.
Frosmo ID cookie workflow
The process for setting the Frosmo ID cookie from your server flows as follows:
-
A new visitor arrives on your site. The initial HTTP request from the visitor's browser to the web server does not contain the
frosmo_uid
cookie. -
The Frosmo Platform loads in the browser and sets the
frosmo_uid
cookie. -
The visitor interacts with the site, resulting in the browser making a new HTTP request to the web server. The request now includes the
frosmo_uid
cookie set by the Frosmo Platform. -
The web server sets the
frosmo_uid_server
cookie in the HTTP response.-
In processing the HTTP request, the web server checks for and finds the
frosmo_uid
cookie. -
The web server creates the
frosmo_uid_server
cookie with the value of thefrosmo_uid
cookie and with expiry set to 1 year from now. -
The web server sends an HTTP response to the browser containing the
frosmo_uid_server
cookie in aSet-Cookie
header.
-
-
The browser stores the
frosmo_uid_server
cookie for the site.
As the visitor continues to interact with the site, both the Frosmo Platform and your server-side applications can now use the frosmo_uid_server
cookie to get the visitor's Frosmo ID.
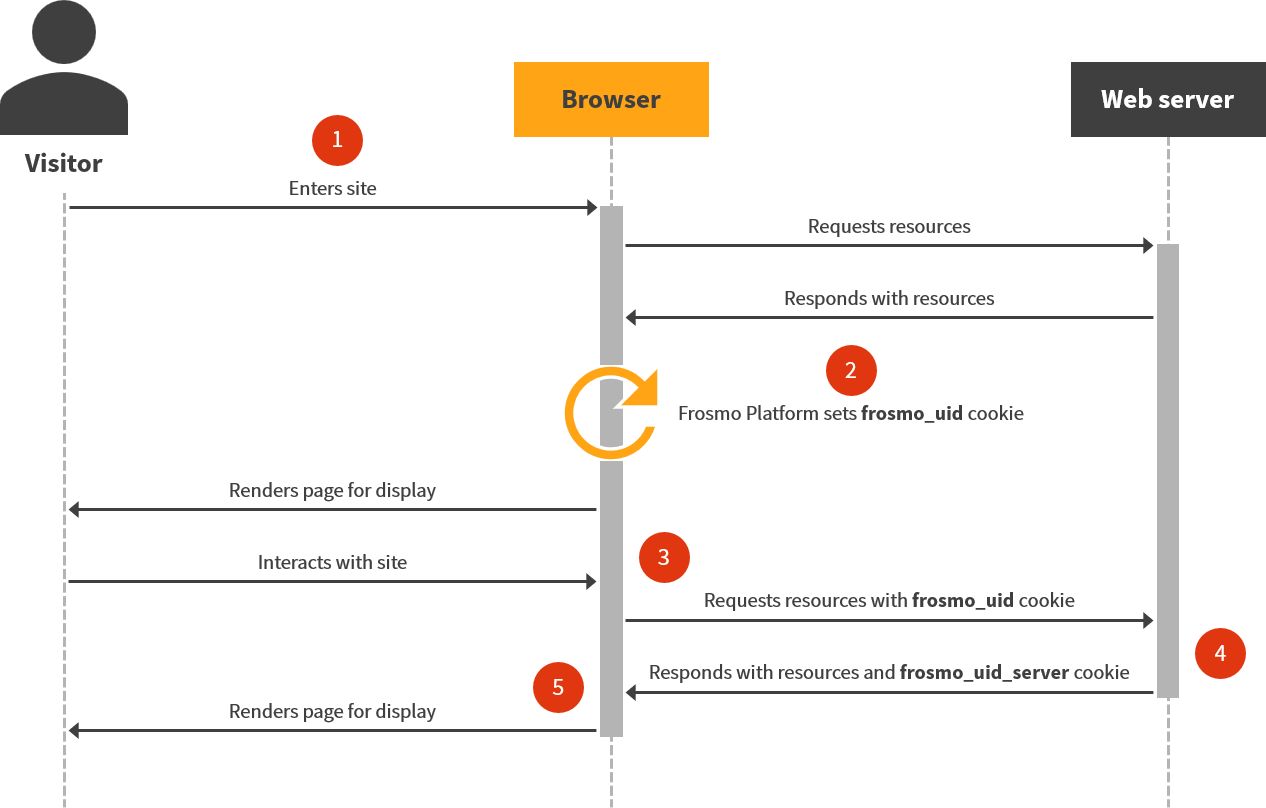
Frosmo ID cookie code examples
The following examples show you how to set the Frosmo ID cookie in a server-side application using common programming languages.
Do not copy and paste an example as is. Instead, adapt the example to your particular code.
func handler(w http.ResponseWriter, r *http.Request) {
frosmoUIDCookie, err := r.Cookie("frosmo_uid")
if errors.Is(err, http.ErrNoCookie) {
// Optionally, handle the case when the cookie missing
return
}
if err != nil {
// Handle the error for all other cases
return
}
http.SetCookie(w, &http.Cookie{
Name: "frosmo_uid_server",
Value: frosmoUIDCookie.Value,
Expires: time.Now().AddDate(1, 0, 0),
HttpOnly: false,
})
// Complete the response...
}
import { parse, serialize } from 'cookie';
function handler(req, res) {
const cookies = parse(req.headers.cookie ?? '');
const frosmoUid = cookies.frosmo_uid;
if (!frosmoUid) {
// Optionally, handle the case when the cookie missing
return;
}
res.setHeader(
'Set-Cookie',
serialize('frosmo_uid_server', frosmoUid, {
expires: new Date(Date.now() + 365 * 24 * 60 * 60 * 1000),
httpOnly: false,
}),
);
// Complete the response...
}
import cookieParser from 'cookie-parser';
import express from 'express';
const app = express();
function setFrosmoIDCookie(req, res, next) {
const frosmoUid = req.cookies.frosmo_uid;
if (!frosmoUid) {
// Optionally, handle the case when the cookie missing
next();
return;
}
res.cookie('frosmo_uid_server', frosmoUid, {
expires: new Date(Date.now() + 365 * 24 * 60 * 60 * 1000),
httpOnly: false,
});
next();
}
app.use(cookieParser());
app.use(setFrosmoIDCookie);
<?php
if (isset($_COOKIE['frosmo_uid'])) {
$frosmoUid = $_COOKIE['frosmo_uid'];
$oneYearFromNow = time() + 365 * 24 * 60 * 60;
setcookie('frosmo_uid_server', $frosmoUid, $oneYearFromNow);
}
// Complete and send the response...
?>
from flask import Flask, request, make_response
import datetime
app = Flask(__name__)
@app.route('/')
def index():
response = make_response("Hello, world!")
frosmo_uid = request.cookies.get('frosmo_uid')
if frosmo_uid:
expires = datetime.datetime.utcnow() + datetime.timedelta(days=365)
response.set_cookie('frosmo_uid_server',
value=frosmo_uid, expires=expires)
return response
Verifying that the Frosmo ID cookie is correctly set
The following instructions reference Google Chrome, but the procedure is basically the same for every modern web browser.
To test that your web server is correctly setting the Frosmo ID cookie:
-
Go to the site.
-
Open the browser's developer tools, and select the Network tab.
-
Reload the page. The Network tab logs every HTTP request that the browser makes during the page load.
-
Select the document request for the current page.
-
In Headers pane, in the Request Headers section, check whether the value of the Cookie field contains both
frosmo_uid
andfrosmo_uid_server
and whether both have the same value.-
If both cookies are present and their values match, the web server is correctly setting the Frosmo ID cookie.
-
If
frosmo_uid_server
is missing or its value does not match thefrosmo_uid
value, there's a problem with your Frosmo ID cookie implementation. Check and fix the implementation or, if needed, contact Frosmo support for further help.
-